In this post I will show how data from WinCC Unified V18 can be brought into Unity with the GraphQL for Unity Pro Asset.
First you need WinCC Unified V18 with the GraphQL Server. The GraphQL server comes out of the box with the new version and should be up and running automatically when you download and start your runtime. You should see a process “WCCILgraphQLServer.exe” in your task manager.
In Unity you have to create a project and download the GraphQL for Unity Pro Asset in the Package Manager.
- Prefab: Drag and drop the WinCC Unified Prefab from the Prefabs folder into your scene.
- Connection: In the properties you have to set your GraphQL Host, optionally the port, the path (typically /graphql), and if you want to have a secured TLS connection (HTTPS, WSS).
- Websocket: If you want to subscribe to tag value changes, then you have to open additionally a Websocket connection. Without the Websocket connection you can still read and write tag values, but a subscription to tag value changes is not possible.
- Authorization: Set the username and password to connect to WinCC Unified (at the time of writing, this user must have the role HMI Administrator).
- Logon: Check the “Logon” checkbox, if you want to start the connection at startup (you can also set this “on-demand” during runtime in your code.
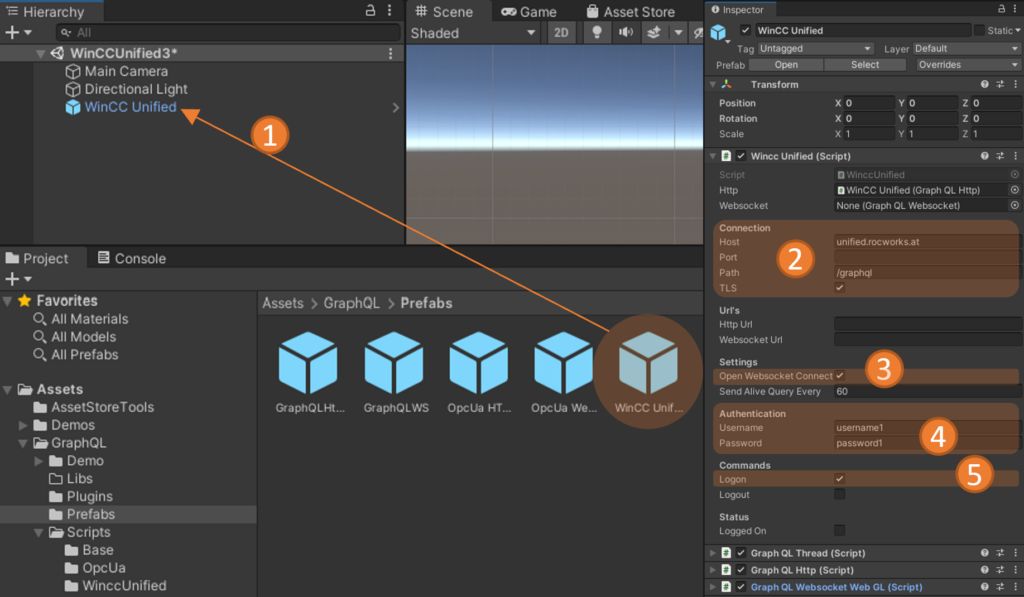
Then you can already start the project in the editor to see if the connection can be established. If everything works fine, then the “Logged On” property turns to checked.
Now you can create your own C# script and read/write/subscribe tag values in an easy way in C# scripting. In that case I have simple added the script as additional component to the WinCC Unified GameObject (Prefab).
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using GraphQLWinccUnified;
using Newtonsoft.Json.Linq;
public class WinccUnified3 : MonoBehaviour
{
private WinccUnified _connection;
private bool _ready;
// Start is called before the first frame update
void Start()
{
_connection = GetComponent<WinccUnified>();
}
// Update is called once per frame
async void Update()
{
if (!_ready && _connection.IsWebsocketReady())
{
_ready = true;
// SubscribeTagValues
_connection.SubscribeTagValues(
new string[] { "HMI_String_1" },
data => { Debug.Log(data.GetValue<string>()); });
// WriteTagValues
await _connection.WriteTagValues(
new string[] { "HMI_String_1" },
new JValue[] { new JValue("Hello World!") },
new DateTime[] { DateTime.Now });
// ReadTagValues
var result = await _connection.ReadTagValues(
new string[] { "HMI_String_1" });
Debug.Log(result[0].GetValue<string>());
}
}
}
If you deal with with a self-signed certificate, then you must uncheck “Validate Certificate” at the “Graph QL Http” Component. But the Websocket connection in Unity does not yet support self-signed certificates. So, it is better to use an insecure connection. Typically the GraphQL port is 4000, so be sure to set the Port to 4000. And don’t forget to open the firewall for that port on the host where the Unified runtime is running.
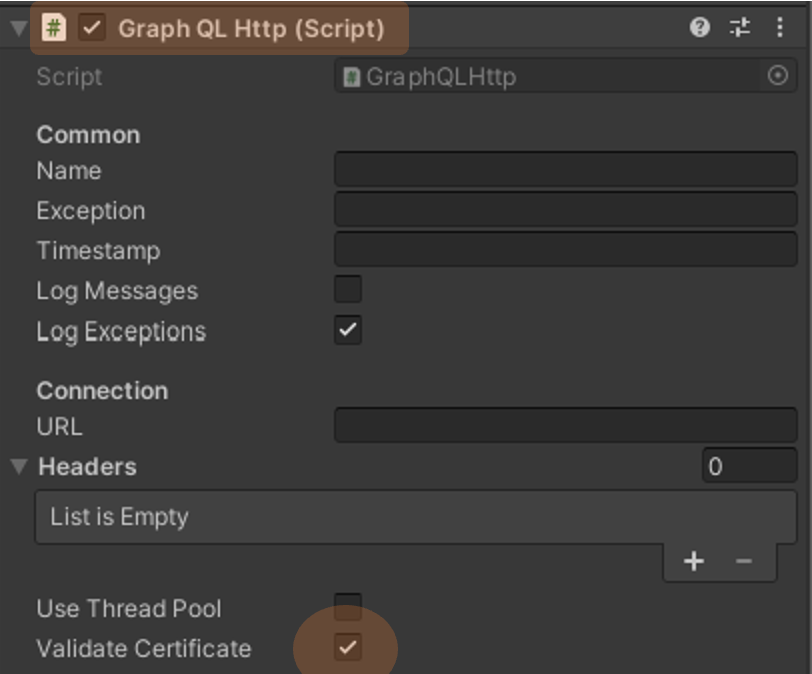